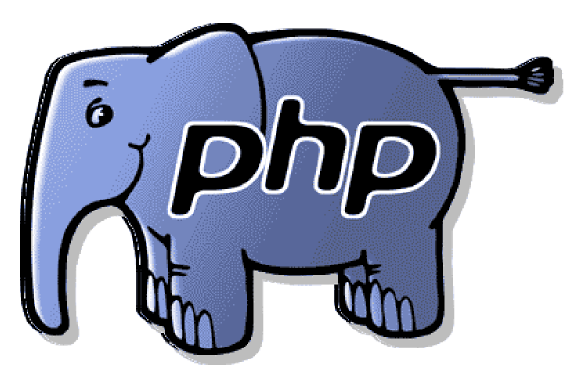
A variable’s type refers to the kind of data that is stored in it.
PHP supports the following data types:
- Integer—Used for whole numbers
- Double—Used for real numbers
- String—Used for strings of characters
- Boolean—Used for true or false values
- Array—Used to store multiple data items of the same type
- Object—Used for storing instances of classes
PHP 4 added three extra types—Boolean, NULL, and resource.Variables that have not
been given a value, have been unset, or have been given the specific value NULL are of type NULL. Certain built-in functions (such as database functions) return variables that have the type resource
Type Casting
You can pretend that a variable or value is of a different type by using a type cast.These work identically to the way they work in C.You simply put the temporary type in brackets in front of the variable you want to cast.
$totalqty = 0;
$totalamount = (double)$totalqty;
Variable Variables
Variable variables enable us to change the name of a variable dynamically.The way these work is to use the value of one variable as the name of another. For
example:
$varname = 'tireqty';
We can then use $$varname in place of $tireqty. For example, we can set the value of
$tireqty:
$$varname = 5;
This is exactly equivalent to
$tireqty = 5;
Constants
As you saw previously, we can change the value stored in a variable.We can also declare constants. A constant stores a value such as a variable, but its value is set once and then cannot be changed elsewhere in the script.You can define these constants using the define function:
define('TIREPRICE', 100);One important difference between constants and variables is that when you refer to a
define('OILPRICE', 10);
define('SPARKPRICE', 4);
constant, it does not have a dollar sign in front of it. If you want to use the value of a
constant, use its name only. For example, to use one of the constants we have just created,
we could type:
echo TIREPRICE;
Operators
Operators are symbols that you can use to manipulate values and variables by performing an operation on them.
Arithmetic operators
Operator Name Example + Addition $a + $b - Subtraction $a - $b * Multiplication $a * $b / Division $a / $b % Modulus $a % $b
String Operators
You can use the string concatenation
operator to add two strings and to generate and store a result much as you would
use the addition operator to add two numbers.
$a = "Bob's ";The $result variable will now contain the string
$b = 'Auto Parts';
$result = $a.$b;
"Bob's Auto Parts"
.Assignment Operators
We’ve already seen
=
, the basic assignment operator.Always refer to this as the assignment operator, and read it as “is set to.” For example,$totalqty = 0;This should be read as “
$totalqty is set to zero
”.Combination Assignment Operators
Operator Use Equivalent to += $a += $b $a = $a + $b -= $a -= $b $a = $a - $b *= $a *= $b $a = $a * $b /= $a /= $b $a = $a / $b %= $a %= $b $a = $a % $b .= $a .= $b $a = $a . $b
Pre- and Post-Increment and Decrement
The pre- and post- increment (++) and decrement (--) operators are similar to the +=
and -= operators, but with a couple of twists.Consider the code below:
$a=4;
echo ++$a;
echo $a;
$a=4;
echo $a++;
echo $a
Output:
5
5
4
5
In case of pre first the value is incremented or decremented then only the echo value is publishes whereas in case of post the value is incremented or decremented only after echo is published.
References
A new addition in PHP 4 is the reference operator, & (ampersand), which can be used in conjunction with assignment. Normally when one variable is assigned to another, a copy is made of the first variable and stored elsewhere in memory. For example,
$a = 5;These lines of code make a second copy of the value in $a and store it in $b. If we subsequently change the value of $a, $b will not change:
$b = $a;
$a = 7; // $b will still be 5You can avoid making a copy by using the reference operator, &. For example,
$a = 5;
$b = &$a;
$a = 7; // $a and $b are now both 7
Comparison Operators
The comparison operators are used to compare two values.
==(equals)
===(identical)
!=(not equal)
<>(not equal)
<(less than)
>(greater than)
<=(less than or equal to)
>=(greater than or equal to)
Logical Operators
The logical operators are used to combine the results of logical conditions. For example,we might be interested in a case where the value of a variable, $a, is between 0 and 100.
! (NOT[eg:!$b (Returns true if $b is false and vice versa)])
&& (AND[eg:$a && $b Returns true if both $a and $b are true; otherwise false])
|| (OR[eg: $a || $b Returns true if either $a or $b or both are true; otherwise false])
and (AND [eg: $a and $b Same as &&, but with lower precedence])
or (OR [eg:$a or $b Same as ||, but with lower precedence])
Bitwise Operators
The bitwise operators enable you to treat an integer as the series of bits used to represent it.You probably will not find a lot of use for these in PHP

Other Operators
Ternary Operator
This operator, ?:, works the same way as it does in C. It takes the form
condition ? value if true : value if falseThe ternary operator is similar to the expression version of an if-else statement which will be discussed later on.
A simple example is
($grade > 50 ? 'Passed' : 'Failed');This expression evaluates student grades to 'Passed' or 'Failed'.
The Error Suppression Operator
The error suppression operator, @, can be used in front of any expression, that is, anything that generates or has a value. For example,
$a = @(57/0);Without the @ operator, this line will generate a divide-by-zero warning (try it).With the operator included, the error is suppressed.
The Execution Operator
The execution operator is really a pair of operators: a pair of backticks (``) in fact.PHP will attempt to execute whatever is contained between the backticks as a command at the command line of the server.The value of the expression is the output of the command.For eg:
$out = `dir c:`;Above script obtain a directory listing and store it in $out. It can then be echoed to the browser or dealt with in any other way.
echo '<pre>'.$out.'</pre>';
to be continued.....................
![]() |
No comments:
Post a Comment