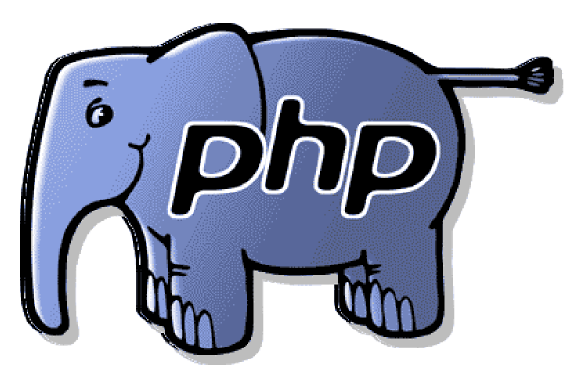
How to write php code
Actually php codes are embedded in the webpage along with the html codes.Remember,if we are embedding php codes in a webpage the page must be saved with .php extension.
A php code must start with <?php and end with ?>.For eg:
<html>
<body>
<?php
echo 'hi';
?>
</body>
</html>
ECHO
echo command is used to print in php.
eg:
<html>
<body>
<?php
echo 'hi';
?>
</body>
</html>
Remember each line of code must end with a ' ; ' as in c/c++ in php.
Defining variables in php
In php there is no need of specifying datatype before variable name.A variable declaration in php takes the following format:
$variablename=value;
A '$' sign followed by variable name.
eg:
<?php
$a=5;
echo $a;
?>
Output of above code will be 5.
Accessing variables from a form
Let us take the example of a signup form in which we are entering values in a textbox.These values are being send to another php page if it is a php website.So there must be a method to take and differentiate values coming from each textboxes in a form and the form must know to which page the values are being send.
We specify the page to which the values are to be send using html code.To be more specific the url to which the values are to be send are specified in the action field of the form tag.The values can be send using GET or POST method.
The difference between GET and POST is that through GET only limited amount of data is sent,it is not secure as data is send through the url.In POST the data send are more secure and there is no limit on the amount of data being send through POST.
Syntax of GET and POST method is:
$_POST['name']
$_GET['name']
where 'name' indicates the name of the field from which the value is to be fetched.
eg:
form.html
<html>
<body>
<form action='index.php?tname='vijay'>
<input type='text' name='uname'/>
<input type='text' name='tname'/>
<input type='submit' value='submit'/>
</form>
</body>
</html>
index.php
<?php
$a=$_POST['uname'];
$b=$_GET['tname'];
echo $a;
echo $b;
?>
In the above example the url in the action field of form tag specifies url of the page to which the data must be sent.'?tname='vijay' along with url indicates the value that is send through GET method.
Now coming to index.php $a gets the value of the field with name='uname' of form.html which is send through POST method.Whereas variable b gets value from variable tname from the url which is sent using GET method.
![]() |
No comments:
Post a Comment