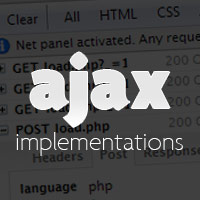
Ajax Script
<script type="text/javascript">
function ajax_request(data,div,file) {
var XHR = null;
if (window.XMLHttpRequest) // Firefox, Safari, Opera, most of browsers.
XHR = new XMLHttpRequest();
else if (window.ActiveXObject) // Internet Explorer
XHR = new ActiveXObject("Microsoft.XMLHTTP");
else { // The browser does not support XMLHttpRequest
alert("Error : Your browser does not support XMLHttpRequest.");
return false;
}
XHR.onreadystatechange = function waiting() {
if (XHR.readyState == 4) {
document.getElementById(div).innerHTML = XHR.responseText;
}
}
XHR.open("POST",file, true);
XHR.setRequestHeader('Content-Type','application/x-www-form-urlencoded');
XHR.send(data);
return false;
}
</script>
Save it as ajaxfunction.js
- The code is not that hard to understand : We create an HttpRequest or ActiveXObject depending of the browser (ActiveXObject is for IE), we send the data via POST, and we get the new informations and write it on the page.
- Well, you don't really need to know how everything works in this function, let's see now the parameters :
function ajax_request(data,div,file)- data : It is the post data which we are going to send to the php script. You have to write it like that : "var1=v1&var2=v2&var3=v3...etc"
- div : It is the container where the response will be written
- file : It is the php file which will be requested for the process.
HTML code
<html>
<head>
<title>Ajax -- Support Forums Tutorial</title>
<script type="text/javascript" src="ajaxfunction.js"></script>
</head>
<body>
<form name="form" onSubmit="ajax_request('promptbox='+document.getElementById ('promptbox').value, 'answer','phpanswer.php');return(false);">
Enter your name > <input type="text" id="promptbox">
</form>
<br />
<div id="answer">
Php is sleeping.
</div>
</body>
</html>
Save it as index.html
php script
<?php
if (isset($_POST['promptbox'])) {
echo "PHP says : Hello ".$_POST['promptbox']." ! *yawns*";
}
?>
Save it as phpanswer.php.
So, let's take a look into the code :
onSubmit="ajax_request('promptbox='+document.getElementById ('promptbox').value, 'answer','phpanswer.php')"
- The first parameter send "promptbox="+the value into the promptbox.
- It will send the data into the div id = "answer"
- And it will request the phpanswer.php file !
You can download the entire script here
No comments:
Post a Comment