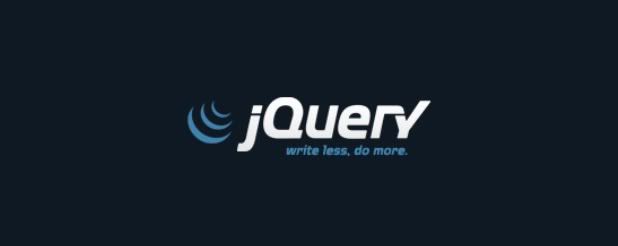
The html page
Let us create a html page which contains a button,on clicking this popup will be displayed.Here is the code for such an html page
<html>
<style>
body
{
margin:0px;
padding:0px;
}
</style>
<body>
<button>click me</button>
</body>
</html>
Container div
When a popup is displayed the user must not be able to edit the web page right? For that we must create a container div that covers the whole webpage.Such div must have height:100%,width:100%,its position:fixed and add opacity:0.7 in its style so that the div would give a translucent effect.
Here is the code for such a div.
<html>
<style>
body
{
margin:0px;
padding:0px;
}
#container
{
position:fixed;
width:100%;
height:100%;
opacity:0.7;
display:none;
background-color:#fff;
}
</style>
<body>
<div id='container'></div>
<button>click me</button>
</body>
</html>
Popup div
Now we shall add a popup div inside the container div.Make its position:absolute.To make it displayed in centre add top:30%;left:35% to its style.Also we have to put a link to close the popup at a the corner.
Here is the code for it
<html>
<style>
body
{
margin:0px;
padding:0px;
}
#container
{
position:fixed;
width:100%;
height:100%;
opacity:0.7;
background-color:#fff;
display:none;
}
#popup
{
position:absolute;
width:200px;
height:200px;
background-color:#000;
top:30%;/*adjust accordingly to position popup*/
left:35%;/*adjust accordingly to position popup*/
/*shadow effect start */
webkit-box-shadow: 0px 1px 5px 0px #4a4a4a;
-moz-box-shadow: 0px 1px 5px 0px #4a4a4a;
box-shadow: 0px 1px 5px 0px #4a4a4a;
/*shadow effect end*/
}
#close
{
left:185px;/*adjust accordingly to position close link*/
text-decoration:none;
color:#fff;
position:absolute;
}
</style>
<body>
<div id='container'><div id='popup'><a id='close' href='#'>X</a>Here is the popup.........</div></div>
<button>click me</button>
</body>
</html>
Jquery code
What we have to do now is to display popup ie the container div on clicking button.Here is the code for it.
<html>
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('button').click(function(){
$('#container').fadeIn('slow');
});
$('#close').click(function(){
$('#container').fadeOut('slow');
});
});
</script>
</head>
<style>
body
{
margin:0px;
padding:0px;
}
#container
{
position:fixed;
width:100%;
height:100%;
opacity:0.7;
background-color:#fff;
display:none;
}
#popup
{
position:absolute;
width:200px;
height:200px;
background-color:#000;
top:30%;/*adjust accordingly to position popup*/
left:35%;/*adjust accordingly to position popup*/
/*shadow effect start*/
webkit-box-shadow: 0px 1px 5px 0px #4a4a4a;
-moz-box-shadow: 0px 1px 5px 0px #4a4a4a;
box-shadow: 0px 1px 5px 0px #4a4a4a;
/*shadow effect end*/
}
#close
{
left:185px;/*adjust accordingly to position close link*/
text-decoration:none;
color:#fff;
position:absolute;
}
</style>
<body>
<div id='container'><div id='popup'><a id='close' href='#'>X</a>Here is the popup.........</div></div>
<button>click me</button>
</body>
</html>
Tweak it and enjoy.............
If you have any doubts or need any clarification ask through comments.
No comments:
Post a Comment